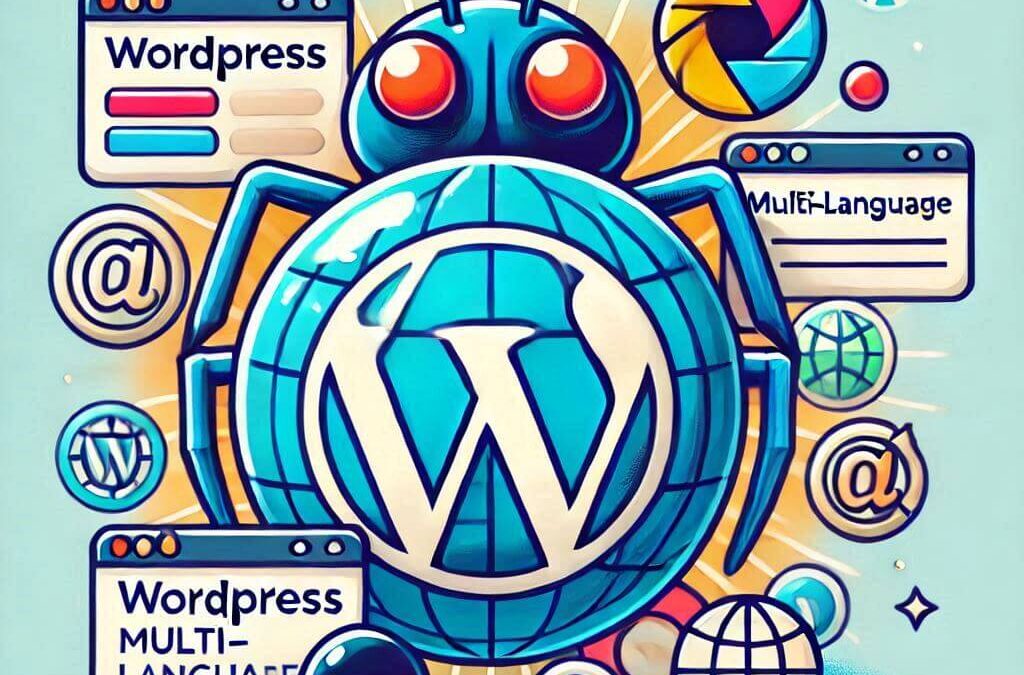
Fixed Function _load_textdomain_just_in_time was called incorrectly
You may encounter this issue in WordPress 6.7:
Function _load_textdomain_just_in_time was called incorrectly.
WordPress now warns developers if they are loading translations too early in a plugin or theme, before the current user is known. Existing functions like load_plugin_textdomain()
and load_theme_textdomain()
were updated to defer the actual loading to the existing just-in-time translation logic in core. This reduces the likelihood of triggering the warning, and even improves performance in some situations by avoiding loading translations if they are not needed on a given page. Here’s how you can fix this issue with straightforward solutions.
Possible Solutions
Use Correct Hooks for Loading Text Domain
If your plugin or theme is calling load_plugin_textdomain
or load_theme_textdomain
functions before WordPress has initialized properly, you need to call these functions on the appropriate hooks like after_setup_theme
or init
.
Here’s an example for each:
add_action( 'init', function () {
load_plugin_textdomain( 'your-textdomain', false, dirname( plugin_basename( __FILE__ ) ) . '/languages/' );
});
For themes
add_action( 'after_setup_theme', function () {
load_theme_textdomain( 'your-textdomain', get_template_directory() . '/languages' );
});
Adjust get_plugin_data
Usage
If you’re using the get_plugin_data
function to read plugin metadata, it might load translations earlier than intended. To prevent this, pass false
as the third argument when calling the function:
$plugin_data = get_plugin_data( __FILE__, true, false );
Debug the Issue
If the problem persists, debugging can help pinpoint the root cause. Add the following snippet to your plugin or theme to track where _load_textdomain_just_in_time
is being called:
add_action(
'doing_it_wrong_run',
static function ( $function_name ) {
if ( '_load_textdomain_just_in_time' === $function_name ) {
debug_print_backtrace();
}
}
);
This snippet will output a stack trace in your debug log whenever _load_textdomain_just_in_time
is called incorrectly. You can use this information to identify and resolve the issue.
Need More Help?
If you’ve tried these solutions and the issue persists, feel free to reach out for assistance. Debugging text domain issues can sometimes require a closer look at your code setup
Let me know in the comments or contact me directly for further support!
I am professional Web Designer & Developer. I have 5+ years of experience in relevant field. Currently I am working for a private software company named “Ollyo” as a Senior Staff Software Engineer.